使用環(huán)境:Windows+python3.4+MySQL5.5+Navicat
一、創(chuàng)建連接
1.準(zhǔn)備工作,,想要使用Python操作MySQL,,首先需要安裝MySQL-Python的包,在Python 3.x下,,該包已經(jīng)改名為MySQLClient,。可以使用pip方式安裝:
或者下載包文件,,進(jìn)行安裝也可以,。
2.Python使用MySQL的流程:

3.啟動MySQL服務(wù)器:以管理員身份啟動“cmd”,輸入命令:’net start mysql‘
Python中使用MySQL導(dǎo)入方法:import MySQLdb
4.創(chuàng)建Connection
Connection:創(chuàng)建了Python客戶端與數(shù)據(jù)庫之間的網(wǎng)絡(luò)通路,。他的參數(shù)如下
參數(shù)名 |
類型 |
說明 |
host |
String |
MySQL的服務(wù)器地址 |
port |
int |
MySQL的端口號 |
user |
String |
用戶名 |
passwd |
String |
密碼 |
db |
String |
使用的數(shù)據(jù)庫 |
charset |
String |
連接字符集 |
Connection支持的方法:
方法名 |
說明 |
cursor() |
創(chuàng)建并且返回游標(biāo) |
commit() |
提交當(dāng)前事物 |
rollback() |
回滾當(dāng)前事物r() |
close() |
關(guān)閉Connection |
5.獲取Cursor.
Cursor:游標(biāo)對象,,用于執(zhí)行查詢和獲取結(jié)果,它支持的方法如下:
方法名 |
說明 |
execute() |
用于執(zhí)行一個數(shù)據(jù)庫的查詢命令 |
fetchone() |
獲取結(jié)果集中的下一行 |
fetchmany(size)
|
獲取結(jié)果集中的下(size)行 |
fetchall() |
獲取結(jié)果集中剩下的所有行 |
rowcount |
最近一次execute返回數(shù)據(jù)/影響的行數(shù) |
close() |
關(guān)閉游標(biāo) |
下面我們在Python中創(chuàng)建一個實例:
import MySQLdb
conn=MySQLdb.connect(host='127.0.0.1',port=3306,user='root',passwd='199331',db='test',charset='utf8')
cursor=conn.cursor()
print(conn)
print(cursor)
cursor.close()
conn.close()
運行程序結(jié)果如下:
從結(jié)果中我們可以看見成功創(chuàng)建了一個Connection和Cursor對象,。
二,、建立數(shù)據(jù)庫,進(jìn)行一些簡單操作
1.簡單的創(chuàng)建一個’user‘表,,并且插入一些數(shù)據(jù),。user表中只有兩個字段:userid和username。代碼如下:
import MySQLdb
conn=MySQLdb.connect(host='127.0.0.1',port=3306,user='root',passwd='199331',db='test',charset='utf8')
cur=conn.cursor()
cur.execute("""
create table if not EXISTS user
(
userid int(11) PRIMARY KEY ,
username VARCHAR(20)
)
""")
for i in range(1,10):
cur.execute("insert into user(userid,username) values('%d','%s')" %(int(i),'name'+str(i)))
conn.commit()
cur.close()
conn.close()
我們用Navicat打開數(shù)據(jù)庫,,查看一下結(jié)果,, ,可以看到成功創(chuàng)建表,,并且插入了十個數(shù)據(jù),。
2.我們操作一下Cursor里面的一些方法。
execute()方法:執(zhí)行SQL,,將一個結(jié)果從數(shù)據(jù)庫獲取到客戶端
fetch*()方法:移動rownumber,,返回數(shù)據(jù)。
例如我們有如下代碼:
1 2 3 4 5 6 7 8 9 10 | sql = 'select * from user'
cursor.execute(sql)
print (cursor.rowcount)
rs = cursor.fetchone()
print (rs)
rs = cursor.fetchmany( 3 )
print (rs)
|
rs=cursor.fetchall()print(rs)
結(jié)果如下:

我們可以看出執(zhí)行查詢?nèi)繑?shù)據(jù)后,,rowcount為10
執(zhí)行fetchone()方法后返回一個數(shù)據(jù),,執(zhí)行fetchmany(3)后返回3條數(shù)據(jù),執(zhí)行fetchall()后返回剩下的所有數(shù)據(jù),。
再有如下代碼:
1 2 3 | res = cursor.fetchall()
for row in res:
print ( 'userid=%s,userna=%s' % row)
|
此時的執(zhí)行結(jié)果為:

3.上面介紹的便是數(shù)據(jù)庫中常說的Select操作,,下面我們介紹數(shù)據(jù)的更新,即:insert,、update,、delete操作。值得注意的是在這部分操作時需要注意的是是否數(shù)據(jù)發(fā)生異常,,如果數(shù)據(jù)沒有發(fā)生異常,,我們便可以直接使用commit()進(jìn)行提交(注:如沒有使用commit,,則數(shù)據(jù)庫不會發(fā)生任何變化)。但是如果出現(xiàn)了異常,,那么久需要使用rollback()進(jìn)行回滾,。
3.1先來看一個沒有異常,正常提交的例子:
1 2 3 4 5 6 7 8 9 10 | sql_insert = 'insert into user(userid,username) values(10,"name10")'
sql_update = 'update user set username="name91" where userid=9'
sql_delete = 'delete from user where userid=3'
cursor.execute(sql_insert)
print (cursor.rowcount)
cursor.execute(sql_update)
print (cursor.rowcount)
cursor.execute(sql_delete)
print (cursor.rowcount)
|
conn.commit()
上面的操作即是:添加一條(10,,’name10‘)的數(shù)據(jù),、將userid=9的username修改為’name91‘,刪除userid=3的數(shù)據(jù),,執(zhí)行上面代碼后我們來用Navicat查看一下數(shù)據(jù):
從結(jié)果可以看到代碼執(zhí)行正常,。
3.2.再來看一個有異常的數(shù)據(jù)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | sql_insert = 'insert into user(userid,username) values(10,"name10")'
sql_update = 'update user set username="name91" where userid=9'
# sql_delete='delete from user where userid=3'
# ##error
sql_delete = 'delete from user where useri=3'
try :
cursor.execute(sql_insert)
print (cursor.rowcount)
cursor.execute(sql_update)
print (cursor.rowcount)
cursor.execute(sql_delete)
print (cursor.rowcount)
except Exception as e:
print (e)
conn.rollback()
|
這里的insert和update操作一樣,只不過把delete里面的userid字段錯誤的寫成了useri,,執(zhí)行代碼:
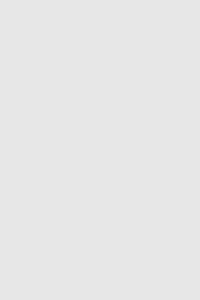
可以看到顯示出異常,,這時我們來看一下數(shù)據(jù)庫數(shù)據(jù):
數(shù)據(jù)沒有任何改變。這就是rollback()的作用
因此,,我們以后再寫增刪改查操作時,,最好把操作放入一個try控制塊中,來避免一些不必要的錯誤,。
下面是一個銀行轉(zhuǎn)賬的實例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | #-*-encoding:utf-8 -*-
import MySQLdb
conn = MySQLdb.connect(host = '127.0.0.1' ,port = 3306 ,user = 'root' ,passwd = '199331' ,db = 'test' ,charset = 'utf8' )
cur = conn.cursor()
##創(chuàng)建數(shù)據(jù)表
cur.execute( """
create table if not EXISTS account(
accid int(10) PRIMARY KEY ,
money int(10)
)
""" )
###插入兩行數(shù)據(jù)
cur.execute( 'insert into account(accid,money) VALUES (1,110)' )
cur.execute( 'insert into account(accid,money) VALUES (2,10)' )
conn.commit()
cur.close()
conn.close()
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 | #-*- encoding:utf-8 -*-
import sys
import MySQLdb
class TransferMoney( object ):
def __init__( self ,conn):
self .conn = conn
def check_acct_available( self ,accid):
cursor = self .conn.cursor()
try :
sql = 'select * from account where accid=%s' % accid
cursor.execute(sql)
print ( 'check_acct_available' + sql)
rs = cursor.fetchall()
if len (rs)! = 1 :
raise Exception( '賬號%s 不存在' % accid)
finally :
cursor.close()
def has_enough_money( self ,accid,money):
cursor = self .conn.cursor()
try :
sql = 'select * from account where accid=%s and money>%s' % (accid,money)
cursor.execute(sql)
print ( 'check_money_available' + sql)
rs = cursor.fetchall()
if len (rs)! = 1 :
raise Exception( '賬號%s 沒有足夠錢' % accid)
finally :
cursor.close()
def reduce_money( self ,accid,money):
cursor = self .conn.cursor()
try :
sql = 'update account set money=money-%s where accid=%s' % (money,accid)
cursor.execute(sql)
print ( 'reduce money' + sql)
rs = cursor.fetchall()
if cursor.rowcount! = 1 :
raise Exception( '賬號%s 減款失敗' % accid)
finally :
cursor.close()
def add_money( self ,accid,money):
cursor = self .conn.cursor()
try :
sql = 'update account set money=money+%s where accid=%s' % (money,accid)
cursor.execute(sql)
print ( 'reduce money' + sql)
rs = cursor.fetchall()
if cursor.rowcount! = 1 :
raise Exception( '賬號%s 加款失敗' % accid)
finally :
cursor.close()
def transfer( self ,source_accid,target_accid,money):
###檢測兩個賬號是否可用
try :
self .check_acct_available(source_accid)
self .check_acct_available(target_accid)
####檢測付款人是否有足夠的錢
self .has_enough_money(source_accid,money)
self .reduce_money(source_accid,money)
self .add_money(target_accid,money)
self .conn.commit()
except Exception as e:
self .conn.rollback()
raise e
if __name__ = = '__main__' :
source_accid = sys.argv[ 1 ]
target_accid = sys.argv[ 2 ]
money = sys.argv[ 3 ]
conn = MySQLdb.connect(host = '127.0.0.1' ,port = 3306 ,user = 'root' ,passwd = '199331' ,db = 'test' ,charset = 'utf8' )
tr_money = TransferMoney(conn)
try :
tr_money.transfer(source_accid,target_accid,money)
except Exception as e:
print ( '出現(xiàn)問題' + str (e))
finally :
conn.close()
|
|