如果想調(diào)用某個(gè)類的某個(gè)方法可以寫成這樣,這個(gè)方法來自NSObject類
C代碼 
-
performSelector:
-
performSelector:withObject:
-
performSelector:withObject:withObject:
實(shí)際調(diào)用
C代碼 
-
[self performSelector:@selector(displayViews) withObject:nil afterDelay:1.0f];
有三個(gè)方法分別是
C代碼 
-
//父視圖
-
[self.view superview]
-
//所有子視圖
-
[self.view subviews]
-
//自身的window
-
self.view.window
循環(huán)一個(gè)視圖下面所有視圖的方法
C代碼 
-
NSArray *allSubviews(UIView *aView)
-
{
-
NSArray *results = [aView subviews];
-
for (UIView *eachView in [aView subviews])
-
{
-
NSArray *riz = allSubviews(eachView);
-
if (riz) {
-
results = [results arrayByAddingObjectsFromArray:riz];
-
}
-
}
-
return results;
-
}
循環(huán)返回一個(gè)APPLICATION里面所有的VIEW
C代碼 
-
// Return all views throughout the application
-
NSArray *allApplicationViews()
-
{
-
NSArray *results = [[UIApplication sharedApplication] windows];
-
for (UIWindow *window in [[UIApplication sharedApplication] windows])
-
{
-
NSArray *riz = allSubviews(window);
-
if (riz) results = [results arrayByAddingObjectsFromArray: riz];
-
}
-
return results;
-
}
找出所有的父視圖
C代碼 
-
// Return an array of parent views from the window down to the view
-
NSArray *pathToView(UIView *aView)
-
{
-
NSMutableArray *array = [NSMutableArray arrayWithObject:aView];
-
UIView *view = aView;
-
UIWindow *window = aView.window;
-
while (view != window)
-
{
-
view = [view superview];
-
[array insertObject:view atIndex:0];
-
}
-
return array;
-
}
UIView提供了大量管理視圖的方法
C代碼 
-
//加一個(gè)視圖到一個(gè)視圖里面
-
addSubview:
-
//將一個(gè)視圖移到前面
-
bringSubviewToFront:
-
//將一個(gè)視圖推送到背后
-
sendSubviewToBack:
-
//把視圖移除
-
removeFromSuperview
-
//插入視圖 并指定索引
-
insertSubview:atIndex:
-
//插入視圖在某個(gè)視圖之上
-
insertSubview:aboveSubview:
-
//插入視圖在某個(gè)視圖之下
-
insertSubview:belowSubview:
-
//交換兩個(gè)位置索引的視圖
-
exchangeSubviewAtIndex:withSubviewAtIndex:
視圖回調(diào)
C代碼 
-
//當(dāng)加入視圖完成后調(diào)用
-
(void)didAddSubview:(UIView *)subview
-
//當(dāng)視圖移動(dòng)完成后調(diào)用
-
(void)didMoveToSuperview
-
//當(dāng)視圖移動(dòng)到新的WINDOW后調(diào)用
-
(void)didMoveToWindow
-
//在刪除視圖之后調(diào)用
-
(void)willRemoveSubview:(UIView *)subview
-
//當(dāng)移動(dòng)視圖之前調(diào)用
-
(void)didMoveToSuperview:(UIView *)subview
-
//當(dāng)視圖移動(dòng)到WINDOW之前調(diào)用
-
(void)didMoveToWindow
給UIView設(shè)置標(biāo)記和檢索視圖
C代碼 
-
myview.tag = 1001;
-
[self.view viewWithTag:1001];
-
(UILable *)[self.view.window viewWithTag:1001];
視圖的幾何特征
C代碼 
-
//框架
-
struct CGPoint {
-
CGFloat x;
-
CGFloat y;
-
};
-
typedef struct CGPoint CGPoint;
-
-
/* Sizes. */
-
-
struct CGSize {
-
CGFloat width;
-
CGFloat height;
-
};
-
typedef struct CGSize CGSize;
-
-
struct CGRect {
-
CGPoint origin;
-
CGSize size;
-
};
-
typedef struct CGRect CGRect;
-
-
-
-
CGRect rect = CGRectMake(0,0,320,480);
-
UIView *view = [[UIView allow]initWithFrame:rect];
-
-
//將String轉(zhuǎn)成CGPoint 如 @”{3.0,2.5}” {x,y}
-
CGPoint CGPointFromString (
-
NSString *string
-
);
-
-
//將String轉(zhuǎn)成CGRect @”{{3,2},{4,5}}” {{x,y},{w, h}}
-
CGRect CGRectFromString (
-
NSString *string
-
);
-
-
//將String轉(zhuǎn)成CGSize @”{3.0,2.5}” {w, h}
-
CGSize CGSizeFromString (
-
NSString *string
-
);
-
-
//CGPoint轉(zhuǎn)成NSString
-
NSString * NSStringFromCGPoint (
-
CGPoint point
-
);
-
-
//CGRect轉(zhuǎn)成NSString
-
NSString * NSStringFromCGRect (
-
CGRect rect
-
);
-
-
//CGSize轉(zhuǎn)成NSString
-
NSString * NSStringFromCGSize (
-
CGSize size
-
);
-
-
//對(duì)一個(gè)CGRect進(jìn)行修改 以這個(gè)的中心來修改 正數(shù)表示更小(縮小) 負(fù)數(shù)表示更大(放大)
-
CGRect CGRectInset (
-
CGRect rect,
-
CGFloat dx,
-
CGFloat dy
-
);
-
-
//判斷兩個(gè)矩形是否相交
-
bool CGRectIntersectsRect (
-
CGRect rect1,
-
CGRect rect2
-
);
-
-
//初始為0的
-
const CGPoint CGPointZero;
-
const CGRect CGRectZero;
-
const CGSize CGSizeZero;
-
-
//創(chuàng)建CGPoint
-
CGPoint CGPointMake (
-
CGFloat x,
-
CGFloat y
-
);
-
//創(chuàng)建CGRect
-
CGRect CGRectMake (
-
CGFloat x,
-
CGFloat y,
-
CGFloat width,
-
CGFloat height
-
);
-
//創(chuàng)建CGSize
-
CGSize CGSizeMake (
-
CGFloat width,
-
CGFloat height
-
);
仿射變換
C代碼 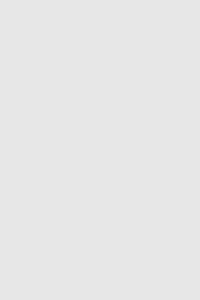
-
CGAffineTransform form = CGAffineTransformMakeRotation(PI);
-
myview.transform = form;
如想復(fù)原
C代碼 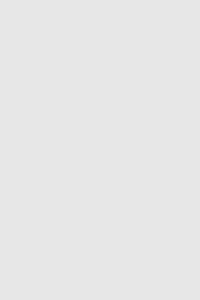
-
myview.transform = CGAffineTransformIdentity;
直接設(shè)置視圖的中心
C代碼 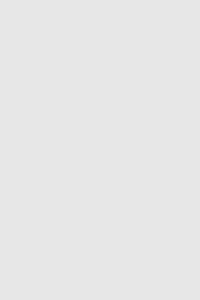
-
myview.center = CGPointMake(100,200);
中心
C代碼 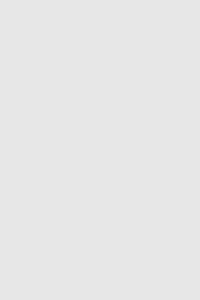
-
CGRectGetMinX
-
CGRectGetMinY
-
//X的中間值
-
CGRectGetMidX
-
//Y的中間值
-
CGRectGetMidY
-
CGRectGetMaxX
-
CGRectGetMaxY
定時(shí)器
C代碼 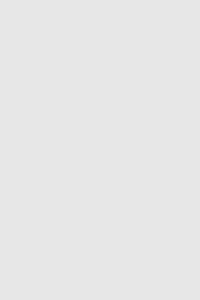
-
NSTime *timer = [NSTimer scheduledTimerWithTimeInterval:0.1f target:self selector:@selector(move:) userInfo:nil repeats:YES];
定義視圖邊界
C代碼 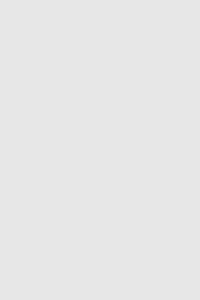
-
typedef struct UIEdgeInsets {
-
CGFloat top, left, bottom, right; // specify amount to inset (positive) for each of the edges. values can be negative to 'outset'
-
} UIEdgeInsets;
-
//eg
-
UIEdgeInsets insets = UIEdgeInsetsMake(5, 5, 5, 5);
-
CGRect innerRect = UIEdgeInsetsInsetRect([aView bounds], insets);
-
CGRect subRect = CGRectInset(innerRect, self.frame.size.width / 2.0f, self.frame.size.height / 2.0f);
仿射變換補(bǔ)充
//創(chuàng)建CGAffineTransform
C代碼 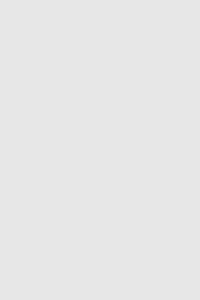
-
//angle 在0-2*PI之間比較好 旋轉(zhuǎn)
-
CGAffineTransform transform = CGAffineTransformMakeRotation(angle);
-
//縮放
-
CGAffineTransform transform = CGAffineTransformMakeScale(0.5f,0.5f);
-
//改變位置的
-
CGAffineTransform transform = CGAffineTransformMakeTranslation(50,60);
-
-
//修改CGAffineTransform
-
//修改 縮放
-
CGAffineTransform scaled = CGAffineTransformScale(transform, degree, degree);
-
//修改 位置
-
CGAffineTransform transform = CGAffineTransformTranslate(
-
CGAffineTransform t,
-
CGFloat tx,
-
CGFloat ty
-
);
-
-
//修改角度
-
CGAffineTransform transform = CGAffineTransformRotate (
-
CGAffineTransform t,
-
CGFloat angle
-
);
-
//最后設(shè)置到VIEW
-
[self.view setTransform:scaled];
建立UIView動(dòng)畫塊
//首先建立CGContextRef
C代碼 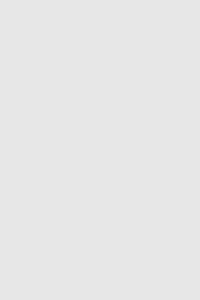
-
CGContextRef context = UIGraphicsGetCurrentContext();
-
//標(biāo)記動(dòng)畫開始
-
[UIView beginAnimations:nil context:context];
-
//定義動(dòng)畫加速或減速的方式
-
[UIView setAnimationCurve:UIViewAnimationCurveEaseInOut];
-
//定義動(dòng)畫的時(shí)長(zhǎng) 1秒
-
[UIView setAnimationDuration:1.0];
-
//中間處理 位置變化,,大小變化,,旋轉(zhuǎn),等等的
-
[[self.view viewWithTag:999] setAlpha:1.0f];
-
//標(biāo)志動(dòng)畫塊結(jié)束
-
[UIView commitAnimations];
-
//還可以設(shè)置回調(diào)
-
[UIView setAnimationDelegate:self];
-
//設(shè)置回調(diào)調(diào)用的方法
-
[UIView setAnimationDidStopSelector:@selector(animationFinished:)];
視圖翻轉(zhuǎn)
C代碼 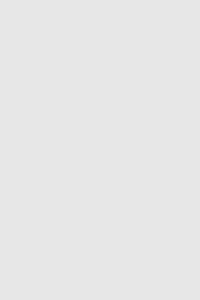
-
UIView *whiteBackdrop = [self.view viewWithTag:100];
-
// Choose left or right flip 選擇左或右翻轉(zhuǎn)
-
if ([(UISegmentedControl *)self.navigationItem.titleView selectedSegmentIndex]){
-
[UIView setAnimationTransition: UIViewAnimationTransitionFlipFromLeft forView:whiteBackdrop cache:YES];
-
}else{
-
[UIView setAnimationTransition: UIViewAnimationTransitionFlipFromRight forView:whiteBackdrop cache:YES];
-
}
-
NSInteger purple = [[whiteBackdrop subviews] indexOfObject:[whiteBackdrop viewWithTag:999]];
-
NSInteger maroon = [[whiteBackdrop subviews] indexOfObject:[whiteBackdrop viewWithTag:998]];
-
//交換視圖
-
[whiteBackdrop exchangeSubviewAtIndex:purple withSubviewAtIndex:maroon];
-
-
//還有上翻和下翻兩種 如下
-
typedef enum {
-
//沒有任何效果
-
UIViewAnimationTransitionNone,
-
UIViewAnimationTransitionFlipFromLeft,
-
UIViewAnimationTransitionFlipFromRight,
-
UIViewAnimationTransitionCurlUp,
-
UIViewAnimationTransitionCurlDown,
-
} UIViewAnimationTransition;
使用QuartzCore做動(dòng)畫
C代碼 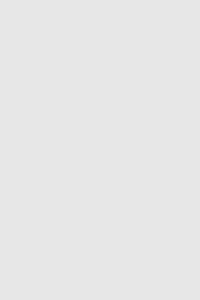
-
//創(chuàng)建CATransition
-
CATransition *animation = [CATransition animation];
-
//設(shè)置代理
-
animation.delegate = self;
-
//設(shè)置動(dòng)畫過渡的時(shí)間
-
animation.duration = 4.0f;
-
//定義動(dòng)畫加速或減速的方式
-
animation.timingFunction = UIViewAnimationCurveEaseInOut;
-
//animation.type 表示設(shè)置過渡的種類 如 Fade,MoveIn,Push,Reveal
-
switch ([(UISegmentedControl *)self.navigationItem.titleView selectedSegmentIndex]) {
-
case 0:
-
animation.type = kCATransitionFade;
-
break;
-
case 1:
-
animation.type = kCATransitionMoveIn;
-
break;
-
case 2:
-
animation.type = kCATransitionPush;
-
break;
-
case 3:
-
animation.type = kCATransitionReveal;
-
default:
-
break;
-
}
-
//設(shè)置漸變的方向,,上下左右
-
if (isLeft)
-
animation.subtype = kCATransitionFromRight;
-
else
-
animation.subtype = kCATransitionFromLeft;
-
-
// Perform the animation
-
UIView *whitebg = [self.view viewWithTag:10];
-
NSInteger purple = [[whitebg subviews] indexOfObject:[whitebg viewWithTag:99]];
-
NSInteger white = [[whitebg subviews] indexOfObject:[whitebg viewWithTag:100]];
-
[whitebg exchangeSubviewAtIndex:purple withSubviewAtIndex:white];
-
[[whitebg layer] addAnimation:animation forKey:@"animation"];
animation.type還可以用以下的賦值
C代碼 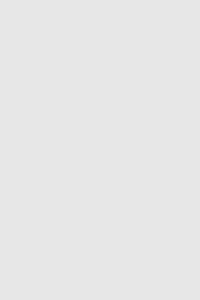
-
switch (theButton.tag) {
-
case 0:
-
animation.type = @"cube";
-
break;
-
case 1:
-
animation.type = @"suckEffect";
-
break;
-
case 2:
-
animation.type = @"oglFlip";
-
break;
-
case 3:
-
animation.type = @"rippleEffect";
-
break;
-
case 4:
-
animation.type = @"pageCurl";
-
break;
-
case 5:
-
animation.type = @"pageUnCurl";
-
break;
-
case 6:
-
animation.type = @"cameraIrisHollowOpen ";
-
break;
-
case 7:
-
animation.type = @"cameraIrisHollowClose ";
-
break;
-
default:
-
break;
-
}
上面這個(gè)是轉(zhuǎn)自這里的http://2015./blog/1122130
休眠一下
C代碼 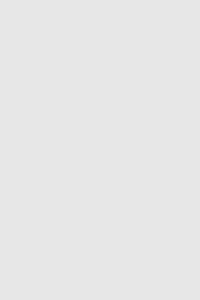
-
[NSThread sleepUntilDate:[NSDate dateWithTimeIntervalSinceNow:1.0f]];
一個(gè)簡(jiǎn)單的通過圖片做的動(dòng)畫
C代碼 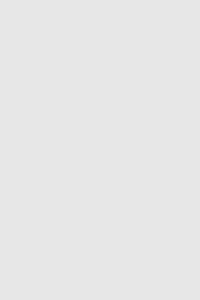
-
// Load butterfly images
-
NSMutableArray *bflies = [NSMutableArray array];
-
for (int i = 1; i <= 17; i++){
-
[bflies addObject:[UIImage imageWithContentsOfFile:[[NSBundle mainBundle] pathForResource:[NSString stringWithFormat:@"bf_%d", i] ofType:@"png"]]];
-
}
-
UIImageView *butterflyView = [[UIImageView alloc] initWithFrame:CGRectMake(40.0f, 300.0f, 60.0f, 60.0f)];
-
butterflyView.tag = 300;
-
//設(shè)置動(dòng)畫的圖片
-
butterflyView.animationImages = bflies;
-
//設(shè)置時(shí)間
-
butterflyView.animationDuration = 0.75f;
-
[self.view addSubview:butterflyView];
-
//開始動(dòng)畫
-
[butterflyView startAnimating];
-
[butterflyView release];
|